Hello everyone,
i have this code on a macos on 2008.
I’m trying to use it now with a windows pc but some problem occurred. (on red the error)
Thanks in advance
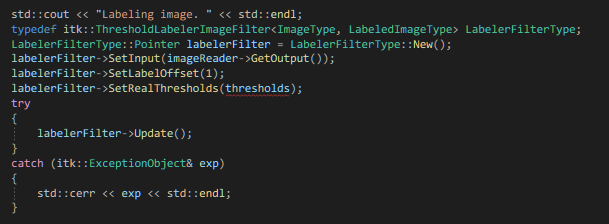
Hello everyone,
i have this code on a macos on 2008.
I’m trying to use it now with a windows pc but some problem occurred. (on red the error)
Thanks in advance
Welcome to the ITK community.
Please provide the text of the error message, as well as copy-pasting the source code instead of posting screenshots of the source code.
this is the code of the part that get the error:
#include <stdio.h>
#include <fstream>
#include "itkImageFileReader.h"
#include "itkImageFileWriter.h"
#include "itkBinaryThresholdImageFilter.h"
#include "itkScalarImageToTextureFeaturesFilter.h"
#include "itkSparseFrequencyContainer2.h"
#include "itkOtsuMultipleThresholdsCalculator.h"
#include "itkThresholdLabelerImageFilter.h"
#include "itkMaskImageFilter.h"
#include "itkImageToListSampleAdaptor.h"
#include "itkSampleToHistogramFilter.h"
#include "itkSparseFrequencyContainer2.h"
#include "itkHistogram.h"
typedef itk::Statistics::ImageToListSampleAdaptor <ImageType> ImageListSample;
typedef itk::Statistics::ImageToListSampleAdaptor <MaskImageType> MaskListSample;
typedef itk::Statistics::SelectiveSubsampleGenerator<ImageListSample, MaskListSample> SubsampleGeneratorType;
typedef itk::Statistics::SampleToHistogramFilter<SubsampleGeneratorType::OutputType, float> HistogramGeneratorType;
ImageListSample::Pointer imageListSample = ImageListSample::New();
imageListSample->SetImage(imageReader->GetOutput());
MaskListSample::Pointer maskListSample = MaskListSample::New();
maskListSample->SetImage(thresholdFilter->GetOutput());
SubsampleGeneratorType::ClassLabelVectorType selectedLabels;
selectedLabels.push_back(itk::NumericTraits<SubsampleGeneratorType::ClassLabelType>::One);
std::cout << "Generating masked subsample" << std::endl;
SubsampleGeneratorType::Pointer subsampleGenerator = SubsampleGeneratorType::New();
subsampleGenerator->SetInput(imageListSample);
subsampleGenerator->SetClassMask(maskListSample);
subsampleGenerator->SetSelectedClassLabels(selectedLabels);
try
{
subsampleGenerator->GenerateData();
}
catch (itk::ExceptionObject& exp)
{
std::cerr << exp << std::endl;
}
std::cout << "Generating histogram" << std::endl;
HistogramGeneratorType::HistogramSizeType numberOfBins;
numberOfBins.Fill(512);
HistogramGeneratorType::Pointer histogramGenerator = HistogramGeneratorType::New();
histogramGenerator->SetListSample(subsampleGenerator->GetOutput());
histogramGenerator->SetNumberOfBins(numberOfBins);
try
{
histogramGenerator->Update();
}
catch (itk::ExceptionObject& exp)
{
std::cerr << exp << std::endl;
}
typedef HistogramGeneratorType::HistogramType HistogramType;
HistogramType::ConstPointer histogram = histogramGenerator->GetOutput();
HistogramType::FrequencyType maxFrequency = itk::NumericTraits<HistogramType::FrequencyType>::min();
HistogramType::MeasurementType mode;
for (HistogramType::ConstIterator iter = histogram->Begin(); iter != histogram->End(); ++iter)
{
if (maxFrequency < iter.GetFrequency())
{
maxFrequency = iter.GetFrequency();
mode = iter.GetMeasurementVector()[0];
}
}
// double lowQuantile = histogram->Quantile(0,0.001);
// double highQuantile = histogram->Quantile(0,0.999);
double lowQuantile = histogram->Quantile(0, 0.01);
double highQuantile = histogram->Quantile(0, 0.99);
// double fatThreshold = 10.0;
if (numberOfClasses == 3 && lowQuantile < fatThreshold)
{
lowQuantile = fatThreshold;
}
HistogramType::Pointer cleanHistogram = HistogramType::New();
HistogramType::SizeType size;
size.Fill(histogram->Size());
HistogramType::MeasurementVectorType lowerBound, upperBound;
HistogramType::IndexType index;
index[0] = 0;
lowerBound[0] = histogram->GetBinMin(0, index[0]);
upperBound[0] = histogram->GetBinMax(0, histogram->Size() - 1);
cleanHistogram->Initialize(size, lowerBound, upperBound);
HistogramType::ConstIterator histiter;
HistogramType::Iterator cleaniter;
for (histiter = histogram->Begin(), cleaniter = cleanHistogram->Begin(); histiter != histogram->End(); ++histiter, ++cleaniter)
{
if ((histiter.GetMeasurementVector()[0] > lowQuantile) && (histiter.GetMeasurementVector()[0] < highQuantile))
{
cleaniter.SetFrequency(histiter.GetFrequency());
}
else
{
cleaniter.SetFrequency(0.0);
}
}
HistogramType::MeasurementType previousMeasurement = histogram->Begin().GetMeasurementVector()[0];
for (HistogramType::ConstIterator iter = histogram->Begin(); iter != histogram->End(); ++iter)
{
int thresholdMarker = 0;
for (unsigned int i = 0; i < numberOfActualClasses - 1; i++)
{
if (thresholds[i] > previousMeasurement && thresholds[i] <= iter.GetMeasurementVector()[0])
{
thresholdMarker = 1;
}
}
dataFileStream << iter.GetMeasurementVector()[0] << "\t" << iter.GetFrequency() << "\t" << thresholdMarker << std::endl;
previousMeasurement = iter.GetMeasurementVector()[0];
}
dataFileStream.close();
}
This is the error when compiled
Your code uses class SelectiveSubsampleGenerator, which has been removed in version 4 of ITK, and therefore does not exist in current, version 5 of ITK either.
You can use old versions of compilers with ITK 3.20, or migrate your code to use newer ITK.
i compiled a itk 3.20, all the classes are recognized but there is one different error:
impossible open ITKStatistics.lib.
Thanks
Did you use CMake to configure your application? If your application is not CMake-based, you can turn it into one by following this hello world example.
Yes I used Cmake to configure!
When asking these kinds of questions, sharing the error message is usually helpful. In this case, sharing your program’s CMakeLists.txt
would be helpful too.
Error messages are best copy-pasted, because that makes them searchable as text (as opposed to screenshots of error messages). Also, text can be copy-pasted into google translate, which would be helpful as I don’t understand Italian.
The cmakelist.txt of the itk is:
the cmakelist.txt of the code is:
cmake_minimum_required(VERSION 3.10.2 FATAL_ERROR)
project(pkdDicomSeriesToVolume)
# Find ITK.
find_package(ITK REQUIRED)
include(${ITK_USE_FILE})
add_executable(pkdDicomSeriesToVolume adpkdotsu.cxx)
target_link_libraries(pkdDicomSeriesToVolume ${ITK_LIBRARIES})
Your CMake code is simplest possible, so I think that the problem is that ITK was not compiled successfully or completely. Can you do an incremental build of ITK?
how can i do an incremental build?
the compile always came with this error:
#error The C++ Standard Library forbids macroizing the keyword “export”.
Enable warning C4005 to find the forbidden define.
On the “Build” menu choose “Build Solution”. That does an incremental build.
You need to build ITK 3.20 with an older compiler. The branch release-3.20
seems like it should build with Visual Studio 2013 and GCC 5.3.1. These commits have been added since 3.20.1 release.
ok ok thanks a lot